Why the shops score so high, and how to fix it. (Algorithms)
Take a good look at this still. I waited until the shop scoring was completed to see what the total was, then I sat down to score myself with pen and paper.
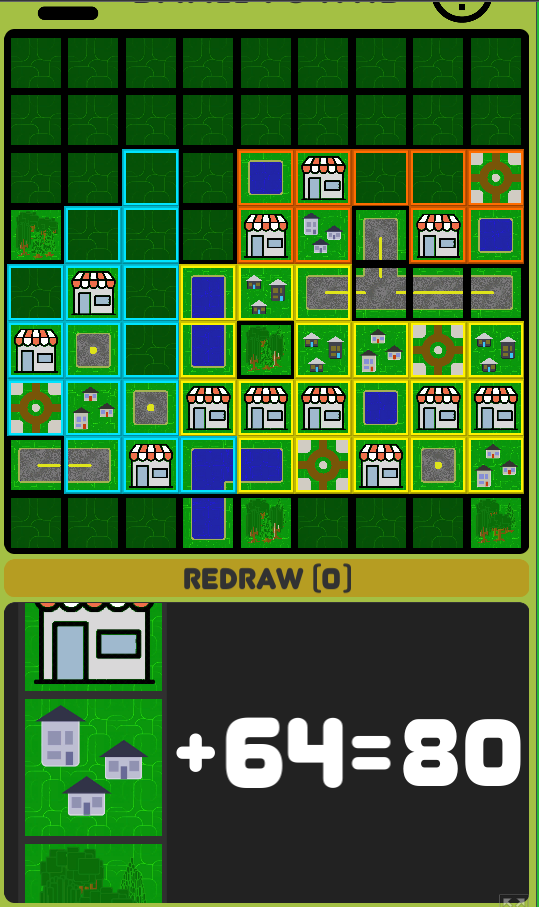
The score I show here is 64 points for shops. That's 80% of the total score so far! The correct score should have been 19. What did I do wrong?
Psuedo Code of my score keeping algorithm for shops: for the purpose of this discussion, adjacent means the fours touching tiles, surrounding means the eight tiles around including the diagonals
foreach (Tile in SurroundingTiles(Shop){ if (Tile.type == House){ score(1); } elseif (Tile.type == Street){ listToCheck.add(Tile); } } while(listToCheck.HasTiles){ if (!listFinished.Contains(listToCheck[0]){ foreach (Tile in AdjacentTiles(listToCheck[0])) { //north, south, east, and west if (Tile.type == House){ score(1); } elseif (Tile.type == Street){ listToCheck.add(Tile); } } listFinished.add(listToCheck[0]); listToCheck.RemoveAt(0); }
Now look at the image above: according to the rules defined above, the shop in square (0, 3) has a house at the diagonal (1,2). It should get one point. but it is also next to a road (1,3), so should be able to reach out to any houses along that road.
The code above does this, but it also incorrectly scores (1,2) again. The shop gets 2 points when it should only get 1. That's a mistake. The same thing happens at (2,1), (5,5), (6,6), so on and so forth. An extra 6 points are added when you do it this way.
But wait, it get's worse:
Let's run that code again, except this time, let's look at tile (7,5). I've highlighted it in this image in white. Along with the 5 houses it should be able to reach.
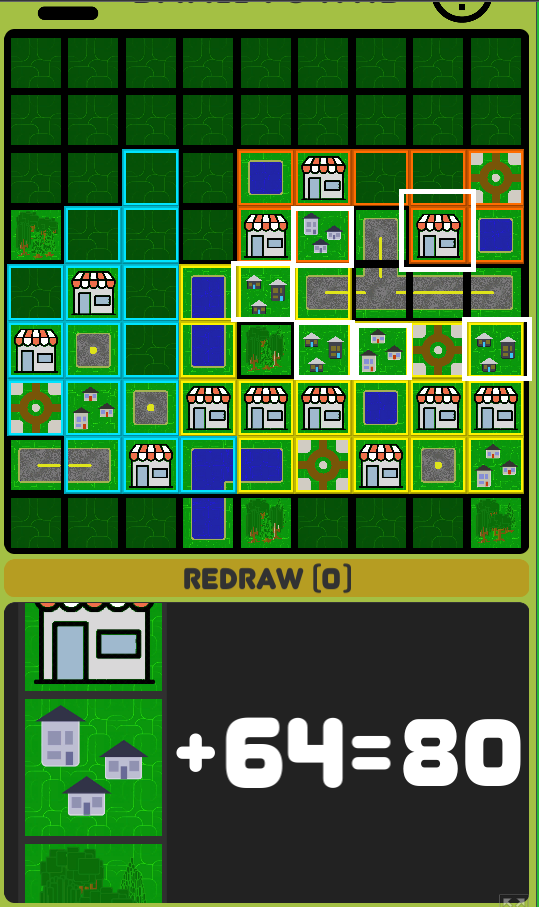
No go back up and run that code again. What do you get? Go ahead I'll wait...
...
...
20, you get 20 points from that shop! Why? Because, even though it doesn't have any surrounding house, it does have 4 street tiles surrounding it. The code written above will walk the road 4 times, scoring the house each time! Ugh...
So, how do I fix this?
I think the answer, is of course is twofold.
1. Shops shouldn't be allowed to access streets from the diagonal.
2. Shops should keep track of where their customers live so they don't count them twice. Maybe give them a discount card so you can track them. That should do it!
But in reality, we just need One More List: scored houses.
foreach (Tile in SurroundingTiles(Shop){ //includes diagonals if (Tile.type == House && !scoredList.Contains(Tile)){ //added a second condition score(1); scoredList.Add(Tile); //add this to our new list } /*elseif (Tile.type == Street){ //removed this and put below listToCheck.add(Tile); }*/ } foreach(Tile in AdjacentTiles(Shop){ //no longer using diagonal tiles if (Tile.type == Street){ listToCheck.add(Tile); } } while(listToCheck.HasTiles){ if (!listFinished.Contains(listToCheck[0]){ foreach (Tile in AdjacentTiles(listToCheck[0])) { //north, south, east, and west if (Tile.type == House && !scoredList.Contains(Tile)) { //again, the second condition score(1); scoredList.add(Tile); } elseif (Tile.type == Street){ listToCheck.add(Tile); } } listFinished.add(listToCheck[0]); listToCheck.RemoveAt(0); }
There, that should do nicely. I can't wait to get home and fix it in game. But for now, my lunchbreak is over. I must return to writing code for "the Man."
Until next time,
Stay Frosty,
~Sub-Zero Chuck
Get Small Towns
Small Towns
A strategy card game where you build small towns
Status | Released |
Author | Sub-Zero Squirrel |
Genre | Card Game, Puzzle, Strategy |
Tags | Board Game, Short, Singleplayer |
More posts
- Polishing Your LookFeb 20, 2022
- Achieving Balance in a Card/Board gameFeb 15, 2022
- Small Towns Is Testing My Resolve.Feb 07, 2022
Leave a comment
Log in with itch.io to leave a comment.